- Selenium Css Selector Cheat Sheet
- Selenium Xpath Cheat Sheet
- Selenium Css Selector Text
- Selenium Selectors Cheat Sheet Pdf
- Selenium Xpath Cheat Sheet Pdf
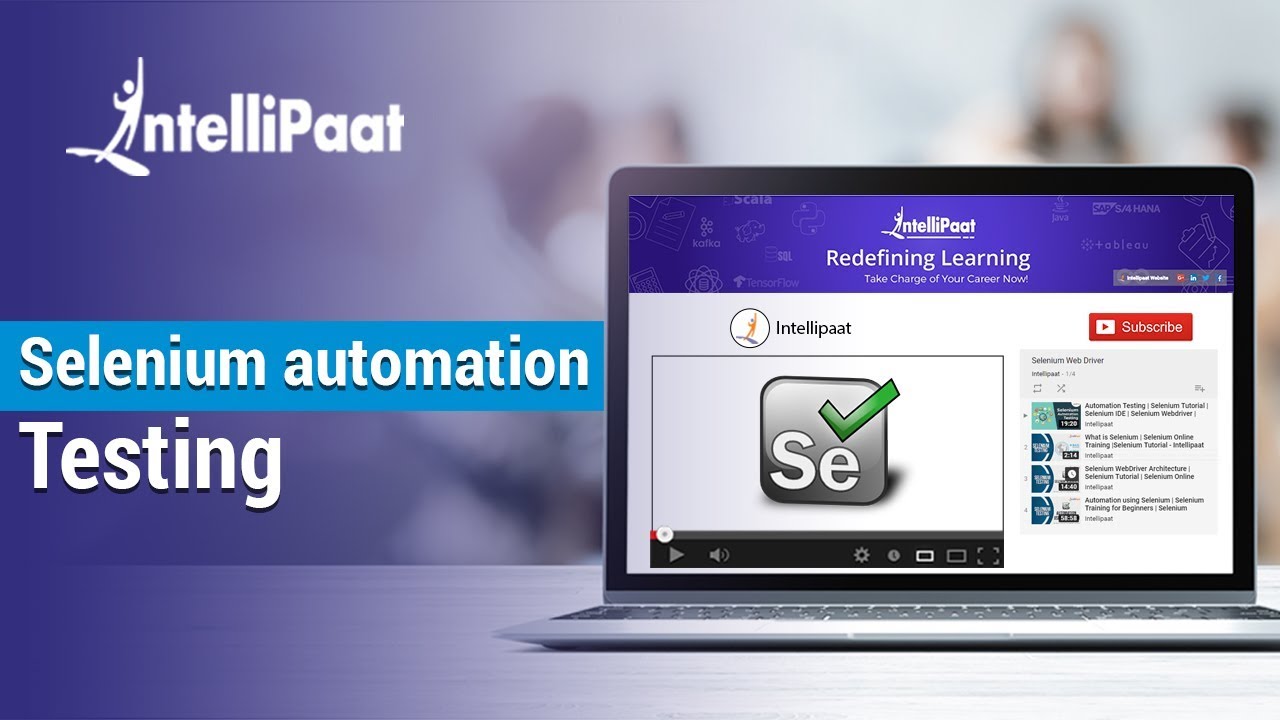
Java selenium commands cheat sheet
Frequently used java selenium commands – Cheat Sheet
- Test Automation For Manual Testers CSS CSS Selectors Cheat Sheet 20:23 Posted by Alex Siminiuc 8 Comments This article presents various CSS expressions for finding web elements.
- Selenium supports 8 different types of locators namely id, name, className, tagName, linkText, partialLinkText, CSS selector and xpath. Using id is one of the most reliable and fast methods of element recognition. Usually, the id is always unique on a given web page. CSS selector and XPath can identify dynamic elements on a web page.
Visit python selenium commands cheat sheet here.
Driver setup:
Firefox:
Frequently used java selenium commands – Cheat Sheet. Visit python selenium commands cheat sheet here. Driver setup: Firefox: System.setProperty(“webdriver.gecko.driver”, “Path To geckodriver”).
System.setProperty(“webdriver.gecko.driver”, “Path To geckodriver”);
To download: Visit GitHub
Chrome:
System.setProperty(“webdriver.chrome.driver”, “Path To chromedriver”);
To download: Visit Here
Internet Explorer:
System.setProperty(“webdriver.ie.driver”, “Path To IEDriverServer.exe”);

To download: Visit Here
Edge:
System.setProperty(“webdriver.edge.driver”, “Path To MicrosoftWebDriver.exe”);
To download: Visit Here
Opera:
System.setProperty(“webdriver.opera.driver”, “Path To operadriver”);
To download: visit GitHub
Safari:
SafariDriver now requires manual installation of the extension prior to automation
Browser Arguments:
–headless
To open browser in headless mode. Works in both Chrome and Firefox browser
–start-maximized
To start browser maximized to screen. Requires only for Chrome browser. Firefox by default starts maximized
–incognito
To open private chrome browser
–disable-notifications

To disable notifications, works Only in Chrome browser
Example:
or
To Auto Download in Chrome:
To Auto Download in Firefox:
We can add any MIME types in the list
Note:
The value of browser.download.folderList can be set to either 0, 1, or 2.
0 – Files will be downloaded on the user’s desktop.
1 – Files will be downloaded in the Downloads folder.
2 – Files will be stored on the location specified for the most recent download
Private browser in Firefox:
Note: you may not see any indication that browser is private. To check, type ‘about:config’ and search for ‘browser.privatebrowsing.autostart’
Disable notifications in Firefox
Read Browser Details:
driver.getTitle();
driver.getWindowHandle();
driver.getWindowHandles();
driver.getCurrentUrl();
driver.getPageSource();
Go to a specified URL:
driver.get(“http://google.com”)
driver.navigate().to(“http://google.com”)
driver.navigate().to(new URL(“http://google.com”))
driver.navigate().back()
driver.navigate().forward()
driver.navigate().refresh()
Locating Elements:
driver.findEelement(By) – To find the first element matching the given locator argument. Returns a WebElement
driver.findElements(By) – To find all elements matching the given locator argument. Returns a list of WebElement
By ID
<input id=”q” type=”text”>…</input>
WebElement element = driver.findElement(By.id(“q”))
By Name
<input id=”q” name=”search” type=”text” /> Hp laserjet m1120 mfp for mac.
WebElement element = driver.findElement(By.name(“search”));
By Class Name
<div class=”username” style=”display: block;”>…</div>
WebElement element = driver.findElement(By.className(“username”));
By Tag Name
<div class=”username” style=”display: block;”>…</div>
WebElement element = driver.findElement(By.tagName(“div”));
By Link Text
<a href=”#”>Refresh</a>
WebElement element = driver.findElement(By.linkText(“Refresh”));
Selenium Css Selector Cheat Sheet
By Partial Link Text
Selenium Xpath Cheat Sheet
<a href=”#”>Refresh Here</a>
WebElement element = driver.findElement(By.partialLinkText(“Refresh”));
By XPath
<form id=”testform” action=”submit” method=”get”>
Username: <input type=”text” />
Password: <input type=”password” />
</form>
WebElement element = driver.findElement(By.xpath(“//form[@id=’testform’]/input[1]”));
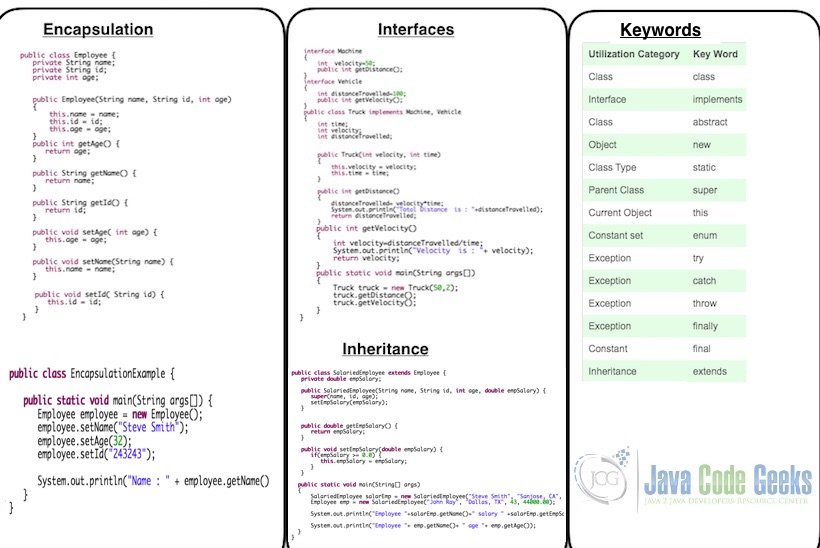
By CSS Selector
<form id=”testform” action=”submit” method=”get”>
<input class=”username” type=”text” />
<input class=”password” type=”password” />
</form>
WebElement element = driver.findElement(By.cssSelector(“form#testform>input.username”));
Java Selenium commands for operation on Elements:
button/link/image:
Selenium Css Selector Text
click()
getAttribute()
isDisplayed()
isEnabled()
Text field:
sendKeys()
clear()
Checkbox/Radio:
isSelected()
click()
Select:
Select select = new Select(WebElement);
select.selectByIndex();
select.selectByValue();
select.selectByVisibleText();
select.deselectAll();
select.deselectByIndex();
select.deselectByValue();
select.deselectByVisibleText();
getFirstSelectedOption()
getAllSelectedOptions() – Returns List
Element properties:
isDisplayed()
isSelected()
isEnabled() Microsoft mouse drivers for mac.
Read Attribute:
getAttribute(“”)
Get attribute from a disabled text box
driver.findElement(By).getAttribute(“value”);
Screenshot:
image storage without using any extra libraries.

Universal Wait:
Wait using jQuery:
Note: jQuery must be defined in Web page otherwise WebDriverException will be thrown. Hence first validate jQuery is defined then check for async calls
The list here contains mostly used java selenium commands but not exhaustive. Please feel free to add in comments if you feel something is missing and should be here.
CSS and XPath can both be used to denote locations in an XML document. These 2 syntaxes help guide your Selenium tests and allow automation to follow your well thought out test strategy. CSS is native to all browsers and specifically built for rendering in browsers, while XPath can give your Selenium test an exactness that is sometimes lacking in CSS. We put together a few tables to help translate XPath to CSS and vice versa. For browser testing, this translation can be especially effective for designers, developers and testers working together during the development and testing phase.
Selenium Selectors Cheat Sheet Pdf
What It Does | XPath | CSS |
---|---|---|
All HTML | /html | html |
Body of Page | /html/body | body |
Element box absolute reference | /body/…/box | body > … > .box |
Any element matching box | box | box |
Any element in the document | * | * |
ID of an element | [@id='green-background'] | #green-background |
Class of an element | [@class='Box'] | .box |
Any element matching title that is a descendant of box | box//title | box > title |
Any element matching title that is a child of box | box/ title | .box .title |
Any element matching title immediately preceded by box element | box/following-sibling::*[1]/self::title | .box + .title |
Image element | //img | img |
Selenium Xpath Cheat Sheet Pdf
What It Does | XPath | CSS |
---|---|---|
Selects first child element | /box/title[1] | .box .title:first-child |
Selects the last child element | /box/title[last()] | .box .title:last-child |
Selects the second to last child element | /box/title[last()-1] | .box .title:nth-last-child(2) |
See Also
Selenium Best Practices
Selenium and Ruby
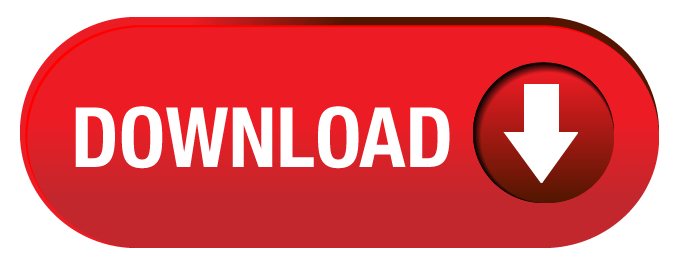